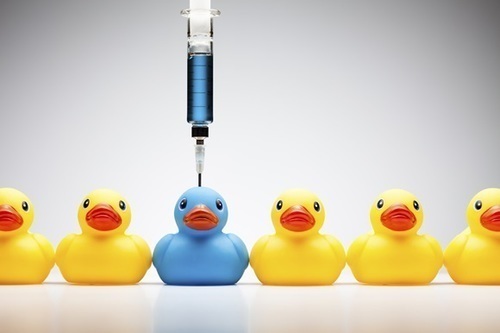
Dependency Injection is a term you may have heard come out of the mouth of the hipster-programmer in front of you in line at a popular chain coffee shop. The term sounds fancier than a sampler plate with seared foie gras, Australian black truffles, and gruyere biscuits. But, what is it? Dependency Injection is a software design pattern where an object’s dependencies are injected through other pieces of code. James Shore gives a concise if not slightly amusing definition in his blog post. "Dependency Injection is a 25-dollar term for a 5-cent concept. […] Dependency injection means giving an object its instance variables.” Wait, is that really it? Basically, yes.
Here is an example of a class that does not use Dependency Injection.
public class MyObject {
private OtherObject otherObject;
public MyObject() {
otherObject = new OtherObject();
}
…
}
Let’s now see this class implemented with basic Dependency Injection.
public class MyObject {
private OtherObject otherObject;
public MyObject(OtherObject otherObject) {
this.otherObject = otherObject;
}
…
}
As you can see, the “dependency”, OtherObject, is “injected” as a parameter to MyObject’s constructor method. What a simple example for such a pretentious sounding programming practice.
Dependency Injection can be used on a much larger scale as well. You loosely-couple different essential components in an application and inject them into each other as needed. An easy way to do this is through what is called interface injection where you basically have an interface and a setter method for a certain component of your application. This gives your application a high level of modularity and flexibility for change. If one component of your application needs to be changed the only other part of your code that would be affected would be what is passed in the setter method linking that component to the rest of your application.
Let’s go over an example of this. Say you have a class, FeedController, that handles a list of Posts provided through some API component. You then create an interface, let’s call it PostProvider, that has a method, getPosts, that returns a list of Posts. The FeedController class would then call a PostProvider’s getPosts method to set the postList.
public class FeedController {
private List<Post> postList;
public FeedController(PostProvider postProvider) {
postList = postProvider.getPosts();
}
…
}
public interface PostProvider {
public List<Post> getPosts();
}
This now allows you to inject different PostProviders to display different Posts from different sources and the FeedController does not care where the Posts comes from.
public class TwitterPostProvider implements PostProvider {
public List<Post> getPosts() {
// Pull tweets…
}
}
public class FacebookPostProvider implements PostProvider {
public List<Post> getPosts() {
// Pull posts…
}
}
Dependency Injection allows the components of an application to be reused with ease. It also removes each component’s responsibility to obtain it’s own resources by delegating tasks to other specialized components. Because of this, components are now loosely-coupled which allows them to be interchanged. There are several Dependency Injecting frameworks available such as Square’s Dagger and Google’s Guice. I encourage you to check them out if you want to learn more.