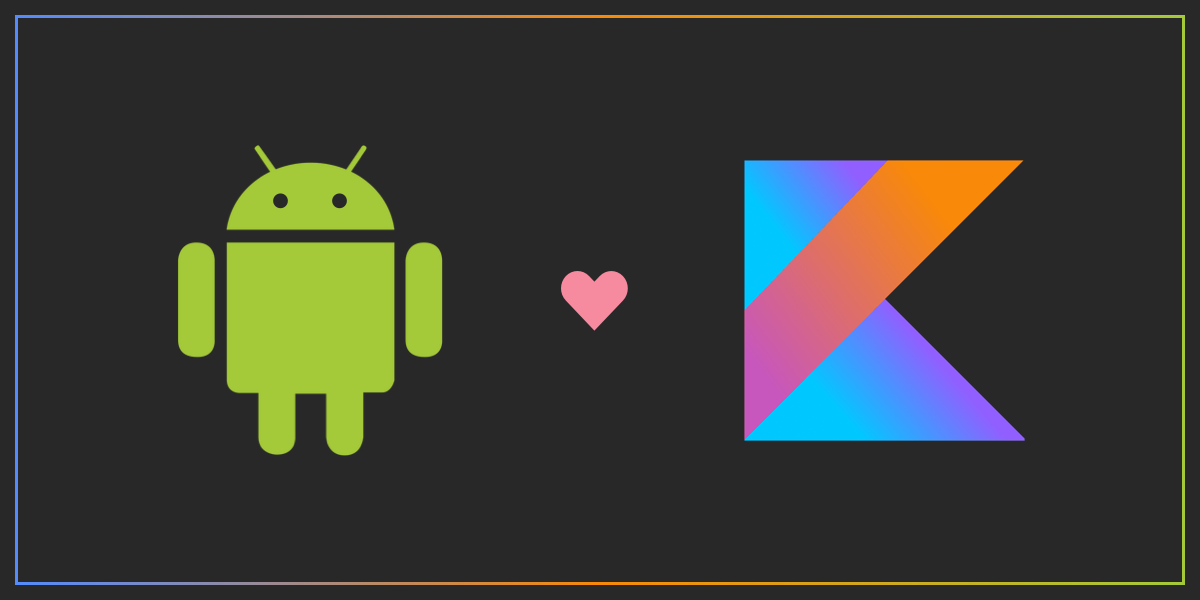
Working at Teeps, I am surrounded by other mobile developers who mostly specialize in iOS. As an Android developer, I find friendly debates with my coworkers over which mobile OS is better an extremely common occurrence in my day-to-day. The most common reason I hear for not wanting to work with Android is Java. This sentiment likely stems from the verbosity of the language requiring more code to complete something than more modern languages, the overall bloated size of the language that has been accumulating over more than two decades, or perhaps simply following the opinions of some trendy development blog. The latter being the most likely reason considering we are talking about iOS developers here! Joking aside, I have to give credit where credit is due, and the introduction of Swift to iOS has left me questioning my early-career decision to focus on Android instead of iOS development.
In steps Kotlin, a shiny beacon of hope!
Kotlin, a statically-typed and open-source language created by IntelliJ IDEA creator, JetBrains, has been around since 2011 and has been gaining popularity in the Android community since the v1.0 stable release in early 2016. Since Kotlin compiles into bytecode it can be used as a direct replacement or supplement to Java for Android development. Kotlin has been used in production at many companies such as Square, Pinterest, and Basecamp. With the announcement at Google IO 2017 for “first-class” support of Kotlin on Android, we can expect a huge surge in companies using Kotlin with Android. There seems to be no better time than now to learn Kotlin, especially considering the learning curve from Java to Kotlin is pretty tame. That said, let’s go over some features Kotlin brings to the table.
Null Safety
A common pitfall when developing on Android with Java is referencing an object when it is null. This throws a NullPointerException (NPE) in Java which anyone who has developed an Android application before has experienced. In Kotlin, if you want to assign a variable to null you have to specifically set specify that it is a nullable type.Here is an example of a variable being defined as a String type.
var a: String = “abc”
If you try to set this variable equal to null you will get a compilation error.
a = null // compilation error
Because of this check at compile time, Kotlin basically eliminates the danger of referencing a null object. Now, if you wanted to explicitly set a variable equal to null you can specify that the variable is nullable by adding a “?”.
var a: String? = “abc”
If we set this variable equal to null we will not see a compilation error.
a = null // okay
Kotlin also offers safe method calling.
b?.length
This will return the length of b if b is not null. If b is null, it will return null instead of throwing a NPE like it would in Java.
You can also set variables using the Elvis Operator.
var l = b?.length ?: -1
This will first check if b is null. If it is not null, l will be set to the length of b. If b is null, the Elvis Operator will catch it and set the value of l to -1.
Slim and Concise Code
One of the major drawbacks of Java is its verbosity. Generally, your classes will tend to be smaller when written in Kotlin than in Java. As you explore the ins and outs of Kotlin, you will notice that your code is easier to read. Let's look at a very simple example of this.
Here we define a Button and add an OnClickListener in Java.
Button button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Do something.
}
}
This will look very familiar to any Android developer. This is cleaned up using libraries such as ButterKnife, but let's look at how this is handled in Kotlin.
val button = findViewById(R.id.button) as Button
button.setOnClickListener {view ->
// Do something.
}
The casting method used and the use of a lambda in Kotlin makes the code easier to read than the Java variant.
First-Class Support
First-class support for Kotlin in Android Studio was announced at Google IO 2017. Previously, Kotlin was usable within Android Studio with the Kotlin plugin. We will see the features provided in this plugin as a standard in Android Studio 3.0. A preview of Android Studio 3.0 can be found here. This isn't as big of a deal as the impact this announcement will have on the Kotlin community. This was basically Google backing Kotlin which will give developers and companies more confidence in using the language in production environments. This means that knowing Kotlin will be a huge bonus to any Android developer's arsenal and resume.
Proper Functional Programming
Although Java 8 brings some functional ability to the table, Java 8 is still not fully supported in Android. Kotlin brings an array of functional power to Android while maintaining all of the procedural power you already expect in Java.
Check back for a future blog post that deep-dives into functional programming in Kotlin as well as several other Kotlin-specific topics tailored to Android developers! In the meantime, here are some resources to further learn about Kotlin.
Resources
Documentation
Books
Courses
Blogs
Example Projects
Are you looking for a team to build your Android app? Fill out our Contact form. We would love to chat.